In this post, I will demonstrate how you can share post on your Facebook Wall or Timeline using Scribe OAuth API and Java.
As a pre-requisite, log into your Facebook account and go to Facebook Developer Apps section. If this is the first time you will have to register (no forms to fill, just a click of a button; more of a permission grant).
Create a new app, just fill the “Basic” information.
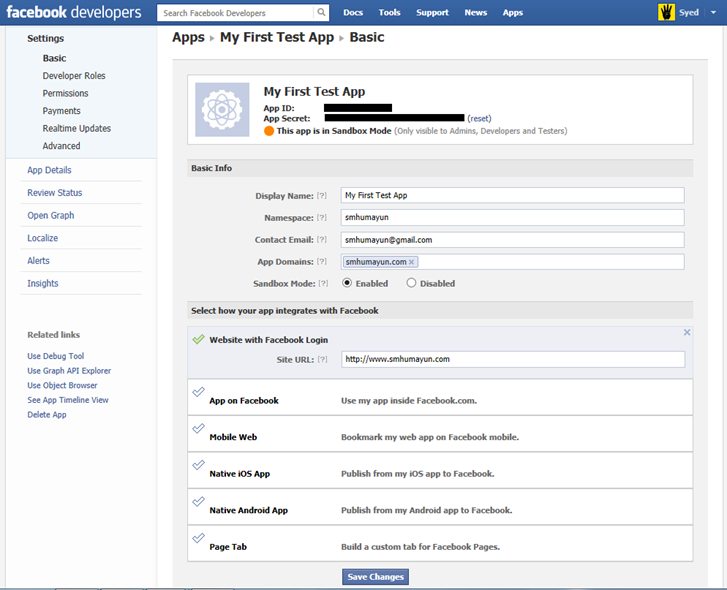
Once you click on “Save Changes”, your new app will be created and a unique “App ID” and “App Secret” will be assigned to your app. YOU SHOULD NOT SHARE THIS WITH ANY ONE and KEEP IT SAFE AND SECURE.
Besides, you will need these (ID & Secret) to make calls to Facebook servers via Graph API.
private static String FB_APP_KEY = "012345678901234";
private static String FB_APP_SECRET = "0123456789abcdef0123456789abcdef";
First thing to do is to initialize Scribe’s OAuthService, so that we can sign our requests to be sent to Facebook servers.
new ServiceBuilder()
.provider(FacebookApi.class)
.apiKey(FB_APP_KEY)
.apiSecret(FB_APP_SECRET)
.callback("http://www.smhumayun.com/”)
.build();
The call back URL used above is the same which we provided as a “Site URL” during our Facebook App creation. With one very important difference, the trailing forward slash “/”. No matter, if you have provided that trailing forward slash in your app’s “Site URL” or not, you MUST always append a forward slash “/” in your callback code. This might seems insane to you, but trust me, a lot of developers have banged their heads against the wall for this minor detail 
Next you’ll have to fetch the Short-Lived Access Token (typically expires in an hour or two).
String authorizationUrl = oAuthService.getAuthorizationUrl(null);
System.out.println(authorizationUrl);
System.out.println("And paste the authorization code here");
System.out.print(">>");
Verifier verifier = new Verifier(new Scanner(System.in).nextLine());
fbAppShortLivedAccessToken = oAuthService.getAccessToken(null, verifier);
The above code will print an Authorzation URL on console, you should copy that URL and open it in a browser and it will redirect to the same “callback” URL (we used above). The redirected URL will contain a ‘code’ query string parameter, copy the value of ‘code’ param and paste it on the console.
If this is a one time test, than you are good with Short-Lived Access Token, but if you want to make it part of a program, you need to exchange it with a Long-Lived Access Token (typically expires in 60 days).
OAuthRequest request = new OAuthRequest(Verb.GET
, "https://graph.facebook.com/oauth/access_token?grant_type=fb_exchange_token"
+ "&client_id=" + FB_APP_KEY + "&client_secret=" + FB_APP_SECRET
+ "&fb_exchange_token=" + fbAppShortLivedAccessToken.getToken());
oAuthService.signRequest(fbAppShortLivedAccessToken, request);
Response response = request.send();
In order to get the Long-Lived Access Token, you have to send the Short-Lived Access Token to Facebook server with an exchange token request with “grant_type” as “fb_exchange_token”. Note, we use the same Short-Lived Access Token to sign this request.
As response to this request, you will get the Long-Lived Access Token, which you can safe/persist and re-use thereafter.
Finally:
OAuthRequest request = new OAuthRequest(Verb.POST, "https://graph.facebook.com/me/feed");
SimpleDateFormat sdf = new SimpleDateFormat("EEE, d MMM yyyy HH:mm:ss Z");
String message = "test message - " + sdf.format(new Date());
request.addBodyParameter("message", message);
String link = "http://codeoftheday.blogspot.com/”;
request.addBodyParameter("link", link);
oAuthService.signRequest(accessToken, request);
You will create a new request to post a ‘message’ and a ‘link’ to your Facebook Wall or Timeline.
If all goes well, and the message/link were posted successfully, you will receive an “id” of the newly created post.